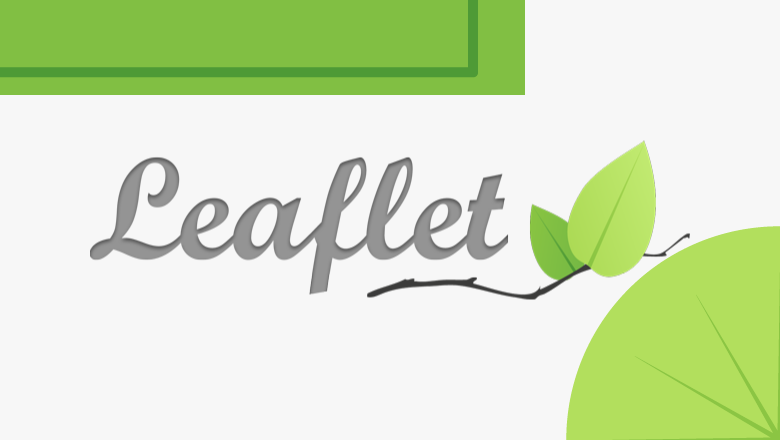
Google Mapsが新プランになったから、Leafletを試してみた
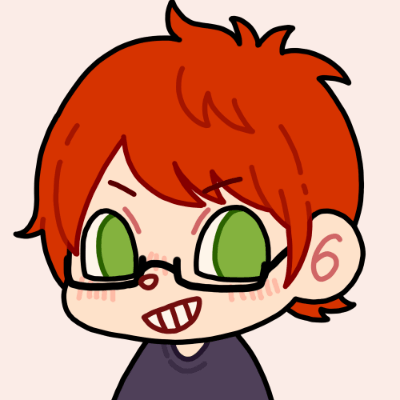
こんにちは。なべっちです。
前回はcreate-react-appを使ったReactの環境構築を行いました。
今回はReactでストップウォッチを作っていきます。
目次
まずは、create-react-appでreact-stopwatchプロジェクトを作成します。
1 |
create-react-app react-stopwatch |
Reactでのスタイリング方法は色々ありますが、今回はstyled-componentsを導入します。
styled-componentsはCSS in JSのライブラリでJSでスタイルを記述します。
1 |
yarn add styled-components |
準備が出来たらアプリケーションを起動します。
1 |
yarn start |
今回はTimer・Button・Appの3つのコンポーネントを組み合わせて作っていきます。
src/Timer.jsを新規作成します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
import React, { Component } from 'react'; import styled from 'styled-components'; class Timer extends Component { constructor() { super(); this.state = { hours: '00', minutes: '00', seconds: '00', time: 0 }; } start() { this.timer = setInterval(() => this.update(), 1000); } stop() { clearInterval(this.timer); } reset() { this.setState({ hours: '00', minutes: '00', seconds: '00', time: 0 }); } update() { const time = this.state.time + 1; const hours = parseInt(time / 60 / 60, 10); const minutes = parseInt(time / 60 % 60, 10); const seconds = parseInt(time % 60, 10); this.setState({ hours: this.toText(hours), minutes: this.toText(minutes), seconds: this.toText(seconds), time: time }); } toText (time) { return ('00' + time).slice(-2); } render() { return( <div> <TimerText>{this.state.hours}</TimerText> <TimerText>:</TimerText> <TimerText>{this.state.minutes}</TimerText> <TimerText>:</TimerText> <TimerText>{this.state.seconds}</TimerText> </div> ); } } export default Timer; const TimerText = styled.span` font-size: 200px; color: #333; ` |
Reactコンポーネントの定義方法には、大きく分けてFunctional ComponentとClass Componentがありますが、Functional Componentでは、stateやrefを使用できません。
stateは、コンポーネントの状態を管理するもので、this.stateで初期値をセットして、this.setStateで更新します。
src/Button.jsを新規作成します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import React from 'react'; import styled from 'styled-components'; const Button = (props) => { return( <Root bgColor={props.bgColor} onClick={() => props.handleClick()}> {props.text} </Root> ); }; export default Button; const Root = styled.button` background-color: ${props => props.bgColor}; width: 100px; height: 50px; font-size: 20px; color: #fff; &:hover { cursor: pointer; } ` |
propsは親から渡される情報です。
Buttonコンポーネントでは、propsによって背景色を変更しています。
src/App.jsを書き換えます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
import React, { Component } from 'react'; import Timer from './Timer'; import Button from './Button'; import styled from 'styled-components'; class App extends Component { render() { return ( <Root> <Title>React Stopwatch</Title> <Timer ref='timer' /> <Unit> <Button text='start' bgColor='#4169e1' handleClick={() => this.refs.timer.start()} /> <Button text='stop' bgColor='#dc143c' handleClick={() => this.refs.timer.stop()} /> <Button text='reset' bgColor='#808080' handleClick={() => this.refs.timer.reset()} /> </Unit> </Root> ); } } export default App; const Root = styled.div` display: flex; flex-direction: column; justify-content: center; align-items: center; width: 100%; height: 100vh; ` const Title = styled.h1` color: #333; ` const Unit = styled.div` display: flex; justify-content: space-between; width: 500px; ` |
Appコンポーネントでは、TimerエレメントとButtonエレメントをimportして、組み合わせます。
Buttonコンポーネントには表示テキストや背景色などをpropsとして渡します。
refはコンポーネントを識別する属性であり、this.refsでそれぞれにアクセスが出来ます。
完成です!お疲れ様でした!
POPULAR
なべっち
Frontend Engineer
人気記事